在MetaTrader平台上,开发者通常使用MQL5语言进行图形对象的操作。以下我们提供了创建、移动和删除等距通道的一些基本函数,以帮助开发者更轻松地在图表上操作通道。
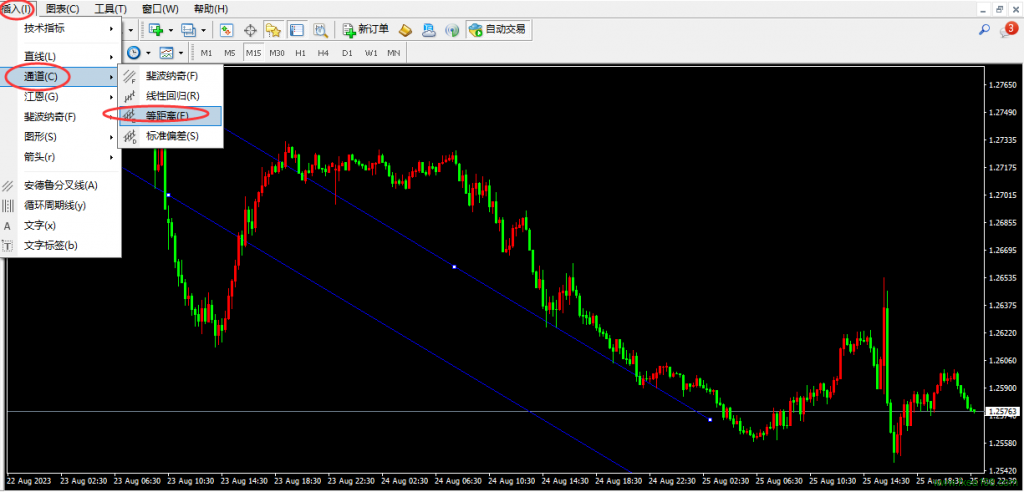
1. 创建等距通道:
bool ChannelCreate(const long chart_ID=0,
const string name="Channel",
const int sub_window=0,
datetime time1=0,
double price1=0,
datetime time2=0,
double price2=0,
datetime time3=0,
double price3=0,
const color clr=clrRed,
const ENUM_LINE_STYLE style=STYLE_SOLID,
const int width=1,
const bool fill=false,
const bool back=false,
const bool selection=true,
const bool ray_right=false,
const bool hidden=true,
const long z_order=0)
{
ChangeChannelEmptyPoints(time1, price1, time2, price2, time3, price3);
ResetLastError();
if(!ObjectCreate(chart_ID, name, OBJ_CHANNEL, sub_window, time1, price1, time2, price2, time3, price3))
{
Print(__FUNCTION__,
": failed to create an equidistant channel! Error code = ", GetLastError());
return(false);
}
ObjectSetInteger(chart_ID, name, OBJPROP_COLOR, clr);
ObjectSetInteger(chart_ID, name, OBJPROP_STYLE, style);
ObjectSetInteger(chart_ID, name, OBJPROP_WIDTH, width);
ObjectSetInteger(chart_ID, name, OBJPROP_BACK, back);
ObjectSetInteger(chart_ID, name, OBJPROP_SELECTABLE, selection);
ObjectSetInteger(chart_ID, name, OBJPROP_SELECTED, selection);
ObjectSetInteger(chart_ID, name, OBJPROP_RAY_RIGHT, ray_right);
ObjectSetInteger(chart_ID, name, OBJPROP_HIDDEN, hidden);
ObjectSetInteger(chart_ID, name, OBJPROP_ZORDER, z_order);
return(true);
}
2. 更改通道的锚点位置:
bool ChannelPointChange(const long chart_ID=0,
const string name="Channel",
const int point_index=0,
datetime time=0,
double price=0)
{
if(!time) time=TimeCurrent();
if(!price) price=SymbolInfoDouble(Symbol(), SYMBOL_BID);
ResetLastError();
if(!ObjectMove(chart_ID, name, point_index, time, price))
{
Print(__FUNCTION__,
": failed to move the anchor point! Error code = ", GetLastError());
return(false);
}
return(true);
}
3. 删除等距通道:
bool ChannelDelete(const long chart_ID=0,
const string name="Channel")
{
ResetLastError();
if(!ObjectDelete(chart_ID, name))
{
Print(__FUNCTION__,
": failed to delete the channel! Error code = ", GetLastError());
return(false);
}
return(true);
}
4. 更改通道空锚点的位置:
void ChangeChannelEmptyPoints(datetime &time1, double &price1, datetime &time2,
double &price2, datetime &time3, double &price3)
{
if(!time2) time2=TimeCurrent();
if(!price2) price2=SymbolInfoDouble(Symbol(), SYMBOL_BID);
if(!time1)
{
datetime temp[10];
CopyTime(Symbol(), Period(), time2, 10, temp);
time1=temp[0];
}
if(!price1) price1=price2+300*SymbolInfoDouble(Symbol(), SYMBOL_POINT);
if(!time3) time3=time1;
if(!price3) price3=price2;
}
以上函数提供了对等距通道的基本操作。